I'm having the same problem that is described here:
www.thewindowsclub.com
But I experience it specifically with black and white images created by my own C# project.
For reference I've posted the code below - it worked fine some years ago but no longer today.
Because I trust my Windows version 11 pro to be up to date and fine, I have this question:
Can I do anything from the C# code in order to fix this?
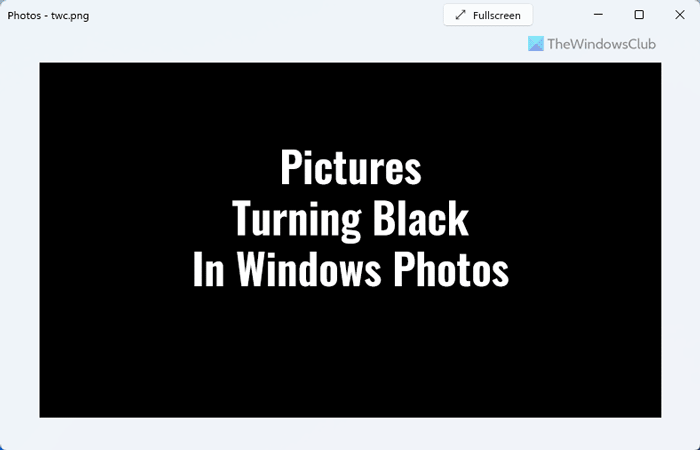
Pictures turning black in Photos app of Windows 11/10
If pictures are turning black after opening in the Windows Photos app, it could be because of app file corruption. Do this to fix the issue.
But I experience it specifically with black and white images created by my own C# project.
For reference I've posted the code below - it worked fine some years ago but no longer today.
Because I trust my Windows version 11 pro to be up to date and fine, I have this question:
Can I do anything from the C# code in order to fix this?
C#:
public void SaveMazeAsImage(string pFileName)
{
int w = (MyMaze.MazeWidth * PixelsPerCell) + (edgemarge * 2);
int h = (MyMaze.MazeHeight * PixelsPerCell) + (edgemarge * 2);
Bitmap MyBitmap;
Graphics g;
MyBitmap = new Bitmap(w, h);
g = Graphics.FromImage(MyBitmap);
Pen blackPen = new Pen(Color.Black, PenWidth);
g.DrawLine(blackPen, edgemarge, edgemarge, edgemarge, h - edgemarge);
g.DrawLine(blackPen, edgemarge, h- edgemarge, w - edgemarge, h - edgemarge);
g.DrawLine(blackPen, w - edgemarge, edgemarge, w - edgemarge, h - edgemarge);
g.DrawLine(blackPen, w - edgemarge, edgemarge, edgemarge, edgemarge);
int i;
int j;
for (i = 0; i < this.MyMaze.MazeWidth; i++)
{
for (j = 0; j < this.MyMaze.MazeHeight; j++)
{
Surrounding MySurrounding = this.CellSurrounding(i, j);
if (MyMaze.CellSetColor[i, j] != MarkColor.White)
{
Rectangle rect = new Rectangle(MySurrounding.leftx + (2 * edgemarge), MySurrounding.topy + (2 * edgemarge), PixelsPerCell - (4 * edgemarge), PixelsPerCell - (4 * edgemarge));
SolidBrush somecolorBrush = new SolidBrush(Color.White);
switch (MyMaze.CellSetColor[i, j])
{
case MarkColor.Blue:
somecolorBrush.Color = Color.Blue;
break;
case MarkColor.Red:
somecolorBrush.Color = Color.Red;
break;
case MarkColor.Green:
somecolorBrush.Color = Color.Green;
break;
case MarkColor.Yellow:
somecolorBrush.Color = Color.Yellow;
break;
}
g.FillRectangle(somecolorBrush, rect);
}
if (i < this.MyMaze.MazeWidth - 1)
{
if (this.MyMaze.MyWallsOfCell[i, j].OpenToRight == false)
{
g.DrawLine(blackPen, MySurrounding.rightx, MySurrounding.bottomy, MySurrounding.rightx, MySurrounding.topy);
}
}
if (j < this.MyMaze.MazeHeight - 1)
{
if (this.MyMaze.MyWallsOfCell[i, j].OpenToTop == false)
{
g.DrawLine(blackPen, MySurrounding.leftx, MySurrounding.topy, MySurrounding.rightx, MySurrounding.topy);
}
}
}
}
MyBitmap.Save(pFileName, ImageFormat.Png);
MyBitmap.Save(pFileName + ".jpg", ImageFormat.Jpeg);
MyBitmap.Save(pFileName + ".bmp", ImageFormat.Bmp);
}