C#:
private void button1_Click(object sender, EventArgs e)
{
if (checkBox1.Checked == true)
{
checkBox2.Checked = false;
checkBox3.Checked = false;
this.pictureBox1.Image = Image.FromFile("images\\1.png");
}
if (checkBox2.Checked == true)
{
checkBox2.Checked = false;
checkBox1.Checked = true;
checkBox3.Checked = true;
this.pictureBox1.Image = Image.FromFile("images\\2.png");
}
if (checkBox3.Checked == true)
{
checkBox2.Checked = false;
checkBox1.Checked = false;
this.pictureBox1.Image = Image.FromFile("images\\3.png");
}
}
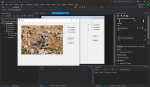
Question is this, I want to be able to check checkbox1 and checkbox2 to show Image 3 then when i want to change the picture I clear it then able to select box 2 and 3 to show image 1, i am a beginner C# .NET forms, and i thought this was correct, but It seems i am doing something wrong in the code. Can somebody help me on what mistake i have taken and show me the right and correct way to get it to work. Thanks.