So, in all of my years of messing with this stuff, I can't think of anything that has made me waste more time than CORS...or been more irritating. I got so tired of it, I have pluggins on Firefox and Chrome that are supposed to bypass CORS, and they aren't working.
I absolutely have traced this down to a CORS issue. If I put a static file in the wwwroot directory of the project, and hit the controller, it works. If I load the same file through a VSCode project w/ different localhost&port, it fails ever time with a CORS error, in Firefox or Chrome.
The following is my setup. If anyone can give me some different ways of troubleshooting this, it would be much appreciated. I have tried many different things, but if you compare to the Microsoft link at the bottom of this post, it looks like it should work to me. Basic troubleshooting has been to change something and reload everything and see my CORS error while debugging.
Thanks...
ConfigureServices(IServiceCollection services)
....
Configure(IApplicationBuilder app, IWebHostEnvironment env)
...
This code matches this Microsoft link:
I absolutely have traced this down to a CORS issue. If I put a static file in the wwwroot directory of the project, and hit the controller, it works. If I load the same file through a VSCode project w/ different localhost&port, it fails ever time with a CORS error, in Firefox or Chrome.
The following is my setup. If anyone can give me some different ways of troubleshooting this, it would be much appreciated. I have tried many different things, but if you compare to the Microsoft link at the bottom of this post, it looks like it should work to me. Basic troubleshooting has been to change something and reload everything and see my CORS error while debugging.
Thanks...
ConfigureServices(IServiceCollection services)
....
C#:
services.AddScoped<ICartService, CartService>();
services.AddCors(options =>
{
options.AddPolicy("MyAllowAllHeadersPolicy",
policy =>
{
policy.WithOrigins("https://localhost:8080")
.WithMethods("PUT", "DELETE", "GET");
});
});
services.AddControllers();
Configure(IApplicationBuilder app, IWebHostEnvironment env)
...
C#:
app.UseRouting();
app.UseCors(); // app.UseCors("MyAllowAllHeadersPolicy");
app.UseAuthorization();
//app.UseCors(x => x.AllowAnyOrigin().AllowAnyHeader().AllowAnyMethod());
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
C#:
namespace WebApplication.Controllers
{
[EnableCors("MyAllowAllHeadersPolicy")]
[Route("api/account")] // [controller]/[action]
[ApiController]
public class AccountController : ControllerBase
This code matches this Microsoft link:
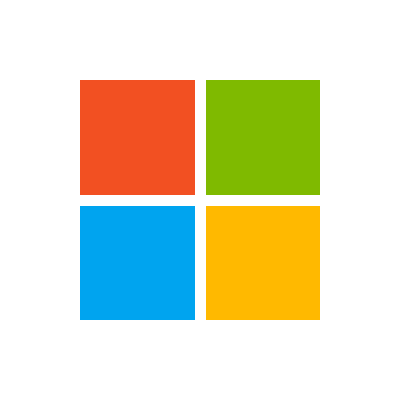
Enable Cross-Origin Requests (CORS) in ASP.NET Core
Learn how CORS as a standard for allowing or rejecting cross-origin requests in an ASP.NET Core app.
docs.microsoft.com