Hi,
I recently wrote some software in C# for a friend, he has been testing it until now. It all works fine, for some minor error in my threading of my class. Will look at that later again and solve it. But for now the biggest issue is File.Move throwing a exception when my download class (fork of monotorrent, which I applied several fixed to..) is finished.
Let me explain a little bit more about how my program works(flow chart).
Inno setup installer > Installing in >%ProgramFiles% > After the installation we run the Installed executable.
Main executable in PF > read local SQLite db, sees first run > Ask for download > YES > Start download thread > Download UI will show up and show details > When done > Move downloaded data from ProgramFiles for example "C:\Program Files (x86)\MyProgram\downloadeddata" to "C:\Program Files (x86)\MyProgram" So it moves ALL files and folder to from downloaddata to the root of the installed application in PF.
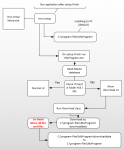
https://i.imgur.com/I0xjDOp.png
Here is where it goes wrong.. When I tested the software on my test machines (Win 7 Pro x64 latest and Win 10 pro x64 latest) it works. But apparently my systems have the default user being administrator.
When My friend tested the software on his system and when he runs the installer it starts my application after Inno is finished, downloads the client data but then when it wants to move all downloaded data it runs in a exception "File x in use". When he ran the application(the installer with run application on finish) as Administrator the exception never showed up.
Why is this? Even on my Win 10 pro system (I did a default install, never messed with it) it works here. I mean UAC and account rights are like default..
Here is the code that does the moving copied from my application.
What is a solution for this or does the application really need administrator rights for this ?
I recently wrote some software in C# for a friend, he has been testing it until now. It all works fine, for some minor error in my threading of my class. Will look at that later again and solve it. But for now the biggest issue is File.Move throwing a exception when my download class (fork of monotorrent, which I applied several fixed to..) is finished.
Let me explain a little bit more about how my program works(flow chart).
Inno setup installer > Installing in >%ProgramFiles% > After the installation we run the Installed executable.
Main executable in PF > read local SQLite db, sees first run > Ask for download > YES > Start download thread > Download UI will show up and show details > When done > Move downloaded data from ProgramFiles for example "C:\Program Files (x86)\MyProgram\downloadeddata" to "C:\Program Files (x86)\MyProgram" So it moves ALL files and folder to from downloaddata to the root of the installed application in PF.
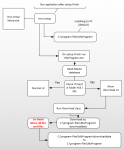
https://i.imgur.com/I0xjDOp.png
Here is where it goes wrong.. When I tested the software on my test machines (Win 7 Pro x64 latest and Win 10 pro x64 latest) it works. But apparently my systems have the default user being administrator.
When My friend tested the software on his system and when he runs the installer it starts my application after Inno is finished, downloads the client data but then when it wants to move all downloaded data it runs in a exception "File x in use". When he ran the application(the installer with run application on finish) as Administrator the exception never showed up.
Why is this? Even on my Win 10 pro system (I did a default install, never messed with it) it works here. I mean UAC and account rights are like default..
Here is the code that does the moving copied from my application.
C#:
public static string RootPath = AppDomain.CurrentDomain.BaseDirectory.ToString();
MoveClient(@RootPath + "downloadeddata\\some-data", @RootPath);
private static void MoveClient(string pobjSource, string pobjTarget)
{
try
{
if (Directory.Exists(pobjTarget) == false)
{
Directory.CreateDirectory(pobjTarget);
}
foreach (string filename in Directory.GetFiles(pobjSource, "*.*"))
{
File.Move(filename, Path.Combine(pobjTarget, Path.GetFileName(filename)));
}
foreach (string objSourceSubDir in Directory.GetDirectories(pobjSource))
{
MoveClient(objSourceSubDir, Path.Combine(pobjTarget, Path.GetFileName(objSourceSubDir)));
}
}
catch (Exception e)
{
MessageBox.Show(e.Message.ToString() + "\r\n" + e.StackTrace + "\r\n" + e.HelpLink + "\r\n" + e.HResult + "\r\n" + e.Source);
}
}
What is a solution for this or does the application really need administrator rights for this ?
Last edited: