Hello, this is my first post. I'm fairly new to C# and specially to file handling, but I've been wanting to code a program that does something like this:
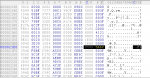
As the picture shows I need to search the file for "05805A6C" and then print to a .txt file the offset "0x21F0".
So far I can only achieve the first step which is loading the file and converting it into a byte array, but have no clue on how to search my array. Could anyone guide some guidance or advice?
Thanks
- Load a file
- Input a start and beginning offset addresses where to scan data from
- Scan that offset range in search of specific sequence of bytes (such as "05805A6C")
- Retrieve the offset of every match and write them to a .txt file
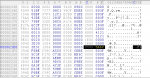
As the picture shows I need to search the file for "05805A6C" and then print to a .txt file the offset "0x21F0".
So far I can only achieve the first step which is loading the file and converting it into a byte array, but have no clue on how to search my array. Could anyone guide some guidance or advice?
Thanks