GTCG
New member
- Joined
- Feb 14, 2016
- Messages
- 2
- Programming Experience
- Beginner
Hey everyone
I've started learning how to program in C# and I have made this:
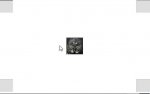
The owl "flies" to the button I click. Like this:
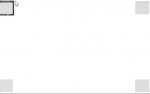
I have made a grid made out of 3 rows and 3 columns.
As you can see, the picture of the owl sits behind the button. How can I change this the other way around? I bet something is wrong with one of the properties of the image or the buttons but I cannot seem to find out what.
My code: ( is in dutch)
Xaml:
Thanks for the help
I've started learning how to program in C# and I have made this:
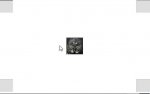
The owl "flies" to the button I click. Like this:
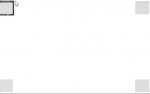
I have made a grid made out of 3 rows and 3 columns.
As you can see, the picture of the owl sits behind the button. How can I change this the other way around? I bet something is wrong with one of the properties of the image or the buttons but I cannot seem to find out what.
My code: ( is in dutch)
C#:
using System;using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace WpfApplication2
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void btnLinksboven_Click(object sender, RoutedEventArgs e) //Bij het klikken op de knop bepalen we de locatie van uil.jpg. Deze moet op rij 0 kolom 0 komen te staan. Dit is ook zo
{ //bij de andere 3 knoppen. Let op. Men begint te tellen vanaf 0. De oefening bestaat uit 3 rijen en 3 kolommen.
imgUil.SetValue(Grid.RowProperty, 0);
imgUil.SetValue(Grid.ColumnProperty, 0);
}
private void btnRechtsboven_Click(object sender, RoutedEventArgs e)
{
imgUil.SetValue(Grid.RowProperty, 0);
imgUil.SetValue(Grid.ColumnProperty, 2);
}
private void btnLinksonder_Click(object sender, RoutedEventArgs e)
{
imgUil.SetValue(Grid.RowProperty, 2);
imgUil.SetValue(Grid.ColumnProperty, 2);
}
private void btnRechtsonder_Click(object sender, RoutedEventArgs e)
{
imgUil.SetValue(Grid.RowProperty, 2);
imgUil.SetValue(Grid.ColumnProperty, 0);
}
}
}
Xaml:
C#:
<Window x:Class="WpfApplication2.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApplication2"
mc:Ignorable="d"
Title="Zie hem vliegen!" Height="350" Width="525">
<Grid x:Name="layoutroot">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="55*"/>
<ColumnDefinition Width="412*"/>
<ColumnDefinition Width="55*"/>
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Height="55*"/>
<RowDefinition Height="235*"/>
<RowDefinition Height="55*"/>
</Grid.RowDefinitions>
<Image x:Name="imgUil" HorizontalAlignment="Center" Height="55" Margin="0" VerticalAlignment="Center" Width="55" Source="uil.jpg" Stretch="Fill" Grid.Row="1" Grid.Column="1"/>
<Button x:Name="btnLinksboven" Content="" Margin="4" Width="55" Height="55" Click="btnLinksboven_Click" BorderThickness="2"/>
<Button x:Name="btnRechtsboven" Content="" Grid.Column="2" Margin="4" Width="55" Height="55" Click="btnRechtsboven_Click"/>
<Button x:Name="btnRechtsonder" Content="" Grid.Column="2" Margin="4" Grid.Row="2" Width="55" Height="55" Click="btnLinksonder_Click"/>
<Button x:Name="btnLinksonder" Content="" Margin="4" Grid.Row="2" Width="55" Height="55" Click="btnRechtsonder_Click"/>
</Grid>
</Window>
Thanks for the help