Hi. I have created loan calculator in C# and want to export the data in listview to pdf, but I don't know how to do it. I am using iTextSharp. I need your help. I am pasting source code here:
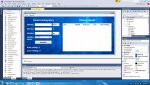
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.IO; using iTextSharp.text; using iTextSharp.text.pdf; namespace Loan_Calculator { public partial class Form1 : Form { public Form1() { InitializeComponent(); this.listView1.ColumnWidthChanging += new ColumnWidthChangingEventHandler(listView1_ColumnWidthChanging); } void listView1_ColumnWidthChanging(object sender, ColumnWidthChangingEventArgs e) { Console.Write("Column Resizing"); e.NewWidth = this.listView1.Columns[e.ColumnIndex].Width; e.Cancel = true; } private void button1_Click(object sender, EventArgs e) { double kreditmeblegi; double illikfaiz; double fm; double value1; double ay; double il; double ayliqodenis; double umumiodenis; double esasborc; double faiz; double qaliqmebleg; if (string.IsNullOrEmpty(textBox1.Text) || string.IsNullOrEmpty(textBox2.Text) || string.IsNullOrEmpty(textBox3.Text)) { MessageBox.Show("Rəqəmləri daxil edin."); } else { if (comboBox2.Text == "ay") { kreditmeblegi = Convert.ToDouble(textBox1.Text); illikfaiz = Convert.ToDouble(textBox2.Text); ay = Convert.ToDouble(textBox3.Text); fm = illikfaiz / (12 * 100); value1 = 1 - (1 / Math.Pow((1 + fm), ay)); ayliqodenis = (kreditmeblegi * fm) / value1; umumiodenis = ayliqodenis * ay; label9.Text = ayliqodenis.ToString("N2") + " " + comboBox1.Text; label10.Text = umumiodenis.ToString("N2") + " " + comboBox1.Text; DateTime date = this.dateTimePicker1.Value.Date; DateTime nyear = new DateTime(); string format = "dd / MM / yyyy"; qaliqmebleg = kreditmeblegi; for (int i = 1; i <= ay; i++) { nyear = date.AddMonths(i); ListViewItem lvi = new ListViewItem(nyear.ToString(format)); lvi.SubItems.Add(ayliqodenis.ToString("N2")); faiz = qaliqmebleg * fm; esasborc = ayliqodenis - faiz; qaliqmebleg = qaliqmebleg - esasborc; lvi.SubItems.Add(esasborc.ToString("N2")); lvi.SubItems.Add(faiz.ToString("N2")); lvi.SubItems.Add(qaliqmebleg.ToString("N2")); listView1.Items.Add(lvi); } button1.Enabled = false; if (Convert.ToDouble(textBox1.Text) == 0 || Convert.ToDouble(textBox2.Text) == 0 || Convert.ToDouble(textBox3.Text) == 0) { MessageBox.Show("Sıfır daxil etmək olmaz!"); button1.Enabled = true; } } else { kreditmeblegi = Convert.ToDouble(textBox1.Text); illikfaiz = Convert.ToDouble(textBox2.Text); il = Convert.ToDouble(textBox3.Text) * 12; fm = illikfaiz / (12 * 100); value1 = 1 - (1 / Math.Pow((1 + fm), il)); ayliqodenis = (kreditmeblegi * fm) / value1; umumiodenis = ayliqodenis * il; DateTime date = this.dateTimePicker1.Value.Date; DateTime nyear = new DateTime(); string format = "dd / MM / yyyy"; qaliqmebleg = kreditmeblegi; for (int j = 1; j <= il; j++) { nyear = date.AddMonths(j); ListViewItem lvi = new ListViewItem(nyear.ToString(format)); lvi.SubItems.Add(ayliqodenis.ToString("N2")); faiz = qaliqmebleg * fm; esasborc = ayliqodenis - faiz; qaliqmebleg = qaliqmebleg - esasborc; lvi.SubItems.Add(esasborc.ToString("N2")); lvi.SubItems.Add(faiz.ToString("N2")); lvi.SubItems.Add(qaliqmebleg.ToString("N2")); listView1.Items.Add(lvi); } label9.Text = ayliqodenis.ToString("N2") + " " + comboBox1.Text; label10.Text = umumiodenis.ToString("N2") + " " + comboBox1.Text; button1.Enabled = false; if (Convert.ToDouble(textBox1.Text) == 0 || Convert.ToDouble(textBox2.Text) == 0 || Convert.ToDouble(textBox3.Text) == 0) { MessageBox.Show("Sıfır daxil etmək olmaz!"); button1.Enabled = true; } } } } private void textBox1_KeyPress(object sender, KeyPressEventArgs e) { if (!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar) && (e.KeyChar != '.')) { e.Handled = true; } // only allow one decimal point if ((e.KeyChar == '.') && ((sender as TextBox).Text.IndexOf('.') > -1)) { e.Handled = true; } } private void textBox2_KeyPress(object sender, KeyPressEventArgs e) { if (!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar) && (e.KeyChar != '.')) { e.Handled = true; } // only allow one decimal point if ((e.KeyChar == '.') && ((sender as TextBox).Text.IndexOf('.') > -1)) { e.Handled = true; } } private void textBox3_KeyPress(object sender, KeyPressEventArgs e) { if (!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar) && (e.KeyChar != '.')) { e.Handled = true; } // only allow one decimal point if ((e.KeyChar == '.') && ((sender as TextBox).Text.IndexOf('.') > -1)) { e.Handled = true; } } private void haqqındaToolStripMenuItem_Click(object sender, EventArgs e) { About about = new About(); about.Show(); } private void wiewHelpToolStripMenuItem_Click(object sender, EventArgs e) { Help melumat = new Help(); melumat.Show(); } private void təmizləToolStripMenuItem_Click(object sender, EventArgs e) { textBox1.Clear(); textBox2.Clear(); textBox3.Clear(); comboBox1.Text = "AZN"; comboBox2.Text = "ay"; dateTimePicker1.Value = DateTime.Now; label9.Text = "0"; label10.Text = "0"; listView1.Items.Clear(); button1.Enabled = true; } private void ?ıxToolStripMenuItem_Click(object sender, EventArgs e) { this.Close(); } private void comboBox1_KeyPress(object sender, KeyPressEventArgs e) { e.Handled = true; } private void comboBox2_KeyPress(object sender, KeyPressEventArgs e) { e.Handled = true; } private void Form1_Load(object sender, EventArgs e) { } private void pDFə?ıxartToolStripMenuItem_Click(object sender, EventArgs e) { FileStream fs = new FileStream("Kreditin Hesablanmasi.pdf", FileMode.Create, FileAccess.Write, FileShare.None); Document doc = new Document(PageSize.A4); PdfWriter writer = PdfWriter.GetInstance(doc, fs); doc.Open(); doc.Add(new Paragraph("Kredit Kalkulyatoru")); doc.Add(new Paragraph("This is a page 2")); doc.Close(); } } }
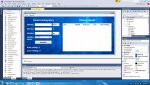
Last edited by a moderator: