spartan.monkey
Member
- Joined
- Oct 25, 2014
- Messages
- 12
- Programming Experience
- Beginner
Hello i want to know if there's any possible way to write all the data that is stored in a c# widows form application to a text file. for example here we have different text boxes and a radio button and other sorts of data. what sort of code would be used if i wanted to save all this data in a .txt file automatically.
secondly we are suppose to allot a roll number to each student which should increase on its own with an increment of 1 (the roll number should appear when the data is stored in a txt file). also that when the text file is being stored the data shouldn't be overwrited.
im a beginner to c#. ive alony made several console applications and this project was assigned to me. please help
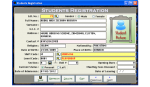
i dont know if this will be usefeul but i googled all day and heres what i found regarding c# writing to a notepad file.
this code was writing the desired textbox to the notepad file but output was like this
System.Windows.Forms.TextBox, Text: HELOELLEOLO.
PLEASSEEEEEE HELP
secondly we are suppose to allot a roll number to each student which should increase on its own with an increment of 1 (the roll number should appear when the data is stored in a txt file). also that when the text file is being stored the data shouldn't be overwrited.
im a beginner to c#. ive alony made several console applications and this project was assigned to me. please help
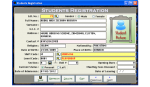
i dont know if this will be usefeul but i googled all day and heres what i found regarding c# writing to a notepad file.
C#:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApplication2
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
// Compose a string that consists of three lines.
string lines = "First line.\r\nSecond line.\r\nThird line.";
string data = "dataaaaaaaaaaaaaaaaaaaaaaa";
// Write the string to a file.
System.IO.StreamWriter file = new System.IO.StreamWriter("d:\\test.txt");
file.WriteLine("{0} {1} {2}", data1,lines,data);
file.Close();
}
}
}
this code was writing the desired textbox to the notepad file but output was like this
System.Windows.Forms.TextBox, Text: HELOELLEOLO.
PLEASSEEEEEE HELP