Socarsky
Well-known member
- Joined
- Mar 3, 2014
- Messages
- 59
- Programming Experience
- Beginner
This is where I want to insert in MS SQL
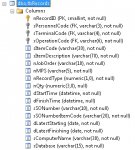
Visual Studio thrown is "Failed to convert parameter value from a DataColumn to a String."
Here is my code for inserting:
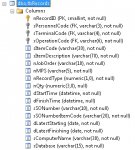
Visual Studio thrown is "Failed to convert parameter value from a DataColumn to a String."
Here is my code for inserting:
private void MSSQLInsert() { newProdID = 0; string ConnStrMSSQL = "Data Source=192.168....;Initial Catalog=xxxx;Persist Security Info=True;User ID=sa;Password=xxxxxx;"; using (SqlConnection con = new SqlConnection(ConnStrMSSQL)) { con.Open(); using (SqlCommand command = new SqlCommand( "INSERT INTO tbRecords VALUES (@sPersonnelCode, @sTerminalCode, " + "@sOperationCode, @sItemCode, @sItemDescription, @nJobOrder, @nMPS, " + "@nRecordType, @nQTY, @dStartTime, @dFinishTime, @sSONumber, @sSONumberItemCode, " + "@dLatestStarting, @dLatestFinishing, @sComputerName, @sDatabase);", con)) { command.Parameters.Add(new SqlParameter("@sPersonnelCode", System.Data.SqlDbType.VarChar, 3)); command.Parameters.Add(new SqlParameter("@sTerminalCode", System.Data.SqlDbType.VarChar, 4)); command.Parameters.Add(new SqlParameter("@sOperationCode", System.Data.SqlDbType.VarChar, 6)); command.Parameters.Add(new SqlParameter("@sItemCode", System.Data.SqlDbType.VarChar, 30)); command.Parameters.Add(new SqlParameter("@sItemDescription", System.Data.SqlDbType.VarChar, 70)); command.Parameters.Add(new SqlParameter("@nJobOrder", System.Data.SqlDbType.VarChar, 10)); command.Parameters.Add(new SqlParameter("@nMPS", System.Data.SqlDbType.VarChar, 5)); command.Parameters.Add(new SqlParameter("@nRecordType", System.Data.SqlDbType.Bit)); command.Parameters.Add(new SqlParameter("@nQTY", System.Data.SqlDbType.Decimal)); command.Parameters.Add(new SqlParameter("@dStartTime", System.Data.SqlDbType.DateTime)); command.Parameters.Add(new SqlParameter("@dFinishTime", System.Data.SqlDbType.DateTime)); command.Parameters.Add(new SqlParameter("@sSONumber", System.Data.SqlDbType.VarChar, 10)); command.Parameters.Add(new SqlParameter("@sSONumberItemCode", System.Data.SqlDbType.VarChar, 20)); command.Parameters.Add(new SqlParameter("@dLatestStarting", System.Data.SqlDbType.Date)); command.Parameters.Add(new SqlParameter("@dLatestFinishing", System.Data.SqlDbType.Date)); command.Parameters.Add(new SqlParameter("@sComputerName", System.Data.SqlDbType.VarChar, 30)); command.Parameters.Add(new SqlParameter("@sDatabase", System.Data.SqlDbType.VarChar, 15)); command.Parameters["@sPersonnelCode"].Value = strPersonnelCode; command.Parameters["@sTerminalCode"].Value = equalDT.Columns["Tezgah"]; command.Parameters["@sOperationCode"].Value = equalDT.Columns["OperationCode"]; command.Parameters["@sItemCode"].Value = equalDT.Columns["ItemCode"]; command.Parameters["@sItemDescription"].Value = equalDT.Columns["ItemDescription"]; command.Parameters["@nJobOrder"].Value = equalDT.Columns["JobOrder"]; command.Parameters["@nMPS"].Value = equalDT.Columns["Evrak"]; command.Parameters["@nRecordType"].Value = 1; command.Parameters["@nQTY"].Value = 1; command.Parameters["@dStartTime"].Value = dateTimeNow(); command.Parameters["@dFinishTime"].Value = dateTimeNow(); command.Parameters["@sSONumber"].Value = equalDT.Columns["SONumber"]; command.Parameters["@sSONumberItemCode"].Value = equalDT.Columns["SONumberItemCode"]; command.Parameters["@dLatestStarting"].Value = equalDT.Columns["LatestStarting"]; command.Parameters["@dLatestFinishing"].Value = equalDT.Columns["LatestFinishing"]; command.Parameters["@sComputerName"].Value = machineName; command.Parameters["@sDatabase"].Value = getDBname; command.ExecuteNonQuery(); // below for seeing colomns in DataTable equalDT //0 takas.Columns.Add(new DataColumn("Tezgah", typeof(string))); //1 takas.Columns.Add(new DataColumn("TezgahAdi", typeof(string))); //2 takas.Columns.Add(new DataColumn("OperationCode", typeof(string))); //3 takas.Columns.Add(new DataColumn("OperationName", typeof(string))); //4 takas.Columns.Add(new DataColumn("ItemCode", typeof(string))); //5 takas.Columns.Add(new DataColumn("ItemDescription", typeof(string))); //6 takas.Columns.Add(new DataColumn("JobOrder", typeof(string))); //7 takas.Columns.Add(new DataColumn("Evrak", typeof(string))); //8 takas.Columns.Add(new DataColumn("QTY", typeof(int))); //9 takas.Columns.Add(new DataColumn("SONumber", typeof(string))); //10 takas.Columns.Add(new DataColumn("SONumberItemCode", typeof(string))); //11 takas.Columns.Add(new DataColumn("LatestStarting", typeof(DateTime))); //12 takas.Columns.Add(new DataColumn("LatestFinishing", typeof(DateTime))); } } }