DotNetDeveloper
New member
- Joined
- Sep 28, 2021
- Messages
- 1
- Programming Experience
- 10+
How to quickly create a PDF document with hierarchical Multilevel List in C#?
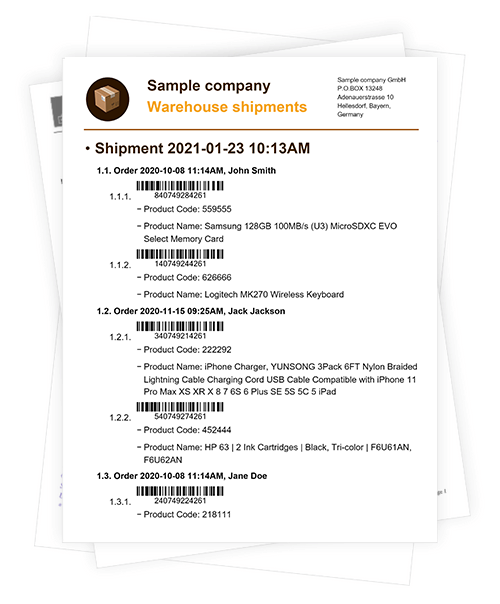
PDF document consists of sections. PDFFlow library for C# allows you to create PDF with as many multilevel lists in one section as you need.Creating a list with PDFFlow is only a few lines of code:
1. First you need to create DocumentBuilder.
2. Then add Section to it.
3. Now add as many Paragraphs to Section, as many list items you need.
4. Make each Paragraph a list item, set level and style if needed.
You can make a paragraph an item of a numbered multilevel list using the ParagraphBuilder.SetListNumbered method. For each item, you can specify the numbering style, the level of the list, the left indent, and enable the hierarchic numeration is necessary. Items of a numbered list are numbered automatically on each level. The available numbering styles are: Arabic numbers, lower and upper Roman numbers, lower and upper Latin letters, lower and upper Cyrillic letters (see the NumerationStyle enumeration).
You can make a paragraph an item of a bulleted multilevel list using the ParagraphBuilder.SetListBulleted method. For each item, you can specify the bullet symbol, the level of the list, and the left indent. The available bullet symbols are a bullet and a dash (see the ListBullet enumeration).
The default level left indent for a multilevel list is 20f, the default numbering style of a numbered list is Arabic, the default bullet symbol is Bullet.
You can configure the formatting settings of list items directly or apply a style to them using methods of ParagraphBuilder. You can also set a style for all lists in the section using the SectionBuilder.SetListStyle method. In this case, you can use the ParagraphBuilder.SetList method to quickly make the necessary paragraphs list items and the set style will be applied to all list items in the section.
Once a paragraph which is not an item of a multilevel list is met, the multilevel list is considered completed.
When later another paragraph is made an item of a multilevel list, it becomes the first item of a new multilevel list, and its automatic numeration will start from the beginning.
Examples
Example 1. Create a numbered list
C#:
using GehtSoft.PDFFlow.Builder;
var latin = NumerationStyle.LowerLatin;
DocumentBuilder.New()
.AddSection()
.AddParagraph("Level 0, Item 1").SetListNumbered()
.ToSection()
.AddParagraph("Level 1, Item a").SetListNumbered(latin, 1)
.ToSection()
.AddParagraph("Level 1, Item b").SetListNumbered(latin, 1)
.ToSection()
.AddParagraph("Level 0, Item 2").SetListNumbered()
.ToSection()
.AddParagraph("Level 0, Item 3").SetListNumbered()
.ToDocument()
.Build("Result.pdf");
The above code will generate the following:
Example 2. Create a bulleted list
C#:
DocumentBuilder.New()
.AddSection()
.AddParagraph("Level 0, Item 1")
.SetFontColor(Color.Red)
.SetListBulleted().ToSection()
.AddParagraph("Level 1, Item 1")
.SetListBulleted(ListBullet.Bullet, 1).ToSection()
.AddParagraph("Level 1, Item 2")
.SetListBulleted(ListBullet.Bullet, 1).ToSection()
.AddParagraph("Level 2, Item 1")
.SetListBulleted(ListBullet.Dash, 2).ToSection()
.AddParagraph("Level 2, Item 2")
.SetListBulleted(ListBullet.Dash, 2).ToSection()
.AddParagraph("Level 0, Item 2")
.SetFontColor(Color.Red)
.SetListBulleted().ToSection()
.AddParagraph("Level 0, Item 3")
.SetFontColor(Color.Red)
.SetListBulleted()
.ToDocument()
.Build("Result.pdf");
The above code will generate the following:
Example 3. Set level indent values
C#:
DocumentBuilder.New()
.AddSection()
.AddParagraph("Level 0, Item 1. Shifted by 5 points")
.SetListBulleted(ListBullet.Bullet, 0, 5)
.ToSection()
.AddParagraph("Level 1, Item 1. Shifted by 15 points")
.SetListBulleted(ListBullet.Bullet, 1, 15)
.ToSection()
.AddParagraph("Level 1, Item 2. Shifted by 15 points")
.SetListBulleted(ListBullet.Bullet, 1, 15)
.ToSection()
.AddParagraph("Level 2, Item 1. Shifted by 20 points")
.SetListBulleted(ListBullet.Dash, 2, 20)
.ToSection()
.AddParagraph("Level 2, Item 2. Shifted by 20 points")
.SetListBulleted(ListBullet.Dash, 2, 20)
.ToDocument()
.Build("Result.pdf");
The above code will generate the following:
Example 4. Set hierarchic numeration
C#:
var latin = NumerationStyle.LowerLatin;
var arabic = NumerationStyle.Arabic;
DocumentBuilder.New().AddSection()
.AddParagraph("Level 0, Item 1")
.SetListNumbered().ToSection()
.AddParagraph("Level 1, Item a")
.SetListNumbered(latin, 1, hierarchicNumeration: true).ToSection()
.AddParagraph("Level 1, Item b")
.SetListNumbered(latin, 1, hierarchicNumeration: true).ToSection()
.AddParagraph("Level 0, Item 2")
.SetListNumbered().ToSection()
.AddParagraph("Level 1, Item 1")
.SetListNumbered(arabic, 1, hierarchicNumeration: true).ToSection()
.AddParagraph("Level 1, Item 2")
.SetListNumbered(arabic, 1, hierarchicNumeration: true).ToSection()
.AddParagraph("Level 0, Item 3")
.SetListNumbered().ToSection()
.AddParagraph("Level 1, Item 1")
.SetListNumbered(arabic, 1, hierarchicNumeration: true).ToSection()
.AddParagraph("Level 1, Item 2")
.SetListNumbered(arabic, 1, hierarchicNumeration: true).ToSection()
.AddParagraph("Level 2, Item 1")
.SetListNumbered(arabic, 2, hierarchicNumeration: true).ToSection()
.AddParagraph("Level 2, Item 2")
.SetListNumbered(arabic, 2, hierarchicNumeration: true).ToSection()
.AddParagraph("Level 1, Item 3")
.SetListNumbered(arabic, 1, hierarchicNumeration: true).ToDocument()
.Build("Result.pdf");
The above code will generate the following:
Example 5. Add a list to a table
C#:
DocumentBuilder.New()
.AddSection()
.AddTable()
.AddColumnToTable().AddColumnToTable()
.AddRow()
.AddCellToRow("Cell1")
.AddCell()
.AddParagraphToCell("List 1:")
.AddParagraph("Item 1").SetListBulleted()
.ToCell()
.AddParagraph("Item 2").SetListBulleted()
.ToCell()
.AddParagraph("Item 3").SetListBulleted()
.ToCell()
.AddParagraphToCell("List 2:")
.AddParagraph("Item 1").SetListNumbered()
.ToCell()
.AddParagraph("Item 2").SetListNumbered()
.ToCell()
.AddParagraph("Item 3").SetListNumbered()
.ToDocument()
.Build("Result.pdf");
The above code will generate the following:
To compile the above examples you need to add Gehtsoft.PDFFlowLib package to your project.
For the installation instructions please read Installing PDFFlow Library article.
The PDFFlow library is supplied with source code samples of real business documents accompanied by descriptive articles.
There are also Tutorials on creating of every PDF element with many working code examples that can be used as is or modified.
Here is a source code and descriptive article of Warehouse Shipment Report, using Multilevel List with hierarchic numeration, barcodes, different fonts, repeating areas and other useful features.
Hope, this article will help you to create complex PDF documents with multilevel lists fast and efficiently.