Hello there!
I am programming something with views, view models, and models, and i need to display a DataGrid. I want to put the names of the colums in a xaml file, so the user can choose a langage package.
I need to declare these colums in my ViewModel file. This work fine when I put the name directly in the header, like this:
But I tried to look at several forums to use my Resource file, and I didn't found anything.
If I modify my code like this:
This doesn't work, here is a screenshot of the result:
As you can see, it's written "System.Windows.DynamicResourceExtension" (basically the name of the class), and it should be written "Actif"
Thanks a lot by advance for your help (and btw, sorry if i did some mistakes in english, i'm French ;p)
I am programming something with views, view models, and models, and i need to display a DataGrid. I want to put the names of the colums in a xaml file, so the user can choose a langage package.
I need to declare these colums in my ViewModel file. This work fine when I put the name directly in the header, like this:
C#:
this.actionColumns.Add(new DataGridCheckBoxColumn {
Header ="Actif", //We define the header
Binding = new Binding("ActifIsChecked"), //We define the Binding
});
If I modify my code like this:
C#:
var resource = new DynamicResourceExtension("actif"); this.actionColumns.Add(new DataGridCheckBoxColumn
{
Header =resource.ToString(), // On definit le header
Binding = new Binding("ActifIsChecked"), // On definit le Binding
});
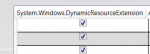
Thanks a lot by advance for your help (and btw, sorry if i did some mistakes in english, i'm French ;p)