Sending SMS using TheTexting SMS Gateway with C#
By Ahmed
Introduction:
In this article, I will walk you through a fast and intriguing approach to send SMS utilizing straightforward C #applications. I make it as simple as feasible for you so I have made both the windows and web demo applications so as to show the guidelines that I will clarify in this article. So simply after taking these simple strides as I walk you through the entire procedure and in the blink of an eye sending SMS will be easy for you.
As cell phones have moved toward becoming a vital part of our regular daily existences and none of us can manage without them anymore, it is not a big surprise that measurements have demonstrated that SMS is the best approach to impact, particularly with regards to a business correspondence. Considering this I set out to demonstrate to you a simple approach to sending SMS using C#.
I will utilize TheTexting SMS API to send SMS, since there are numerous different SMS entryways, you can experiment with any of them by utilizing their free trial account. I could not find any article showing sending SMS using TheTexting gateway, that force me to write an article on sending SMS via TheTexting SMS gateway.
Different ways of sending text messages?
There are different ways of sending text messages like below?
1-Using GSM Modem:
This would be the best decision in the event that you need to send SMS from disconnected applications and in the event that you need to send few SMS for consistently then utilizing GSM modem is the best decision.
2-Using endpoints:
It is the best decision when the quantity of SMS should have been sent surpasses a hundred every moment.
3-Using web service:
This would be the best decision for an online application and you want to send very few messages every minute.
Sending SMS via web service:
We should begin without delving further into the superfluous insights about different SMS API providers in the market. Going into profundities at this stage will just make it additionally befuddling and it is not my expectation to give you a migraine. I will explain the procedure well-ordered with the goal that you can digest easily.
Part one:
Get Started:
The first thing you need to do is to create an account on http://www.thetexting.com so what you need to do is to click on this link while holding the CTRL key of your keyboard. You can also copy and paste the link into your browser window and hit enter key of your keyboard. You will see the below screen.
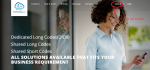
By clicking on the sign up link you will be redirected to the following page as show in below figure
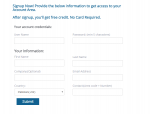
You have to fill out this form with the required information and submit it, after submission of the form you will received SMS as well as email from TheTexting,com and you have to verify both of them before using TheTexting SMS gateway. You can see in the figure bellow how it looks like.
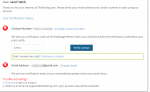
After verification of your phone number and email you would be redirected to the following page as shown below.
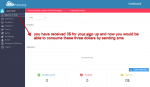
This Dashboard gives you the overall details like your balance, API Settings, profile, and developer references, the one which is of our interest is API Settings, we can generate new API key as well by clicking the Generate API Key button. Just click on API setting and it will show your key and secret values as shown below in figure.
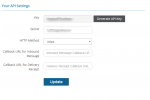
Service URL:
https://www.thetexting.com/rest/sms/json/message/send?api_key=wwpiub1oipk49a1&api_secret=j5hsuac8877mdzdd&to=923218829981&text=Hello"
This is the API service URL which our Web client will be consuming. We just need to change the values of API KEY , SECRET and TO values and hit enter the SMS will be sent to the number which you specified in the To parameter. Is not that a magic without creating any application just with a URL you are able to send SMS? Wow
Now, let?s start with the demo application I am creating WPF application for demonstration for windows platform , if you are unfamiliar with WPF then go to the below link to learn WPF I do not want to spin my article out to teach you WPF first.
https://msdn.microsoft.com/en-us/library/aa970268(v=vs.100).aspx
Here we have four textboxes for four input parameters like API key, secret, To and Message, I have already demonstrated these four parameters above. To get your API key and secret values just login to http://www.thetexting.com and then click on API settings here you will find your API key and Secret values, To is the number to which you want to send SMS and do not forget to include country Code like I did for United sates it is 1. Message is the message which you want to send, due to trial account you cannot provide a custom message TheTexting will send the default message same as you cannot provide the from parameter input value as it also would be by default thetexting itself.
C # code for sending SMS:
public class Response
{
public string message_id { get; set; }
public int message_count { get; set; }
public double price { get; set; }
}
public class RootObject
{
public Response Response { get; set; }
public string ErrorMessage { get; set; }
public int Status { get; set; }
}
private void button1_Click(object sender, RoutedEventArgs e)
{
string key = txtKey.Text;
string secret = txtSecret.Text;
string to = txtTo.Text;
string messages = txtMessage.Text;
string sURL;
sURL = "https://www.thetexting.com/rest/sms/json/message/send?api_key=" + key + "&api_secret=" + secret + "&to=" + to + "&text=" + messages;
try
{
using (WebClient client = new WebClient())
{
string s = client.DownloadString(sURL);
var responseObject = Newtonsoft.Json.JsonConvert.DeserializeObject<RootObject>(s);
int n=responseObject.Status;
if (n == 3)
{
MessageBox.Show("Something went wrong with your credentials", "My Demo App");
}
else
{
MessageBox.Show("Message Send Successfully", "My Demo App");
}
}
}
catch (Exception ex)
{
MessageBox.Show("Something went wrong with your information", "My Demo App");
ex.ToString();
}
}
}
Here we have a very simple code. We have created four variables and we are assigning the four text boxes values to these four variables. Next we are creating a string variable of URL and giving it our service URL and including our parameters values with it i.e. SECRET,API KEY , TO and Message. With this URL{this URL is basically the address of our service}, then we are using web client to download the data, our service will return data in JSON format so now we want to read this JSON result using our c# code,for that we again have two options to do that, one method is to read data from datatable by first pulling the data into the data table and then reading from it, but the best approach is to deserialize JSON data, so we have created two class Response and RootObject and they have their own properties like message id,price,count,status, then we are using Newtonsoft.Json.JsonConvert.DeserializeObject<RootObject>(s) to convert the JSON data to C# and reading the status value to show the specified message to the user i.e. if the status code is 3 then it means that authentication failed and based on these codes we are showing specific messages to the user on screen as message pop-up.
If you need details about how to Deserialize JSON Data then visit below link.
http://stackoverflow.com/questions/7895105/deserialize-json-with-c-sharp
Namespaces Required:
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using System.IO;
using System.Net;
using System.Data;
using System.Xml;
using Newtonsoft.Json;
Note: you must include Newtonsoft.Json.dll into your references, the demo application having already included Newtonsoft.Json.dll but for double check, I have also placed it in zip folder along with the attachments.
Part Two:
How to send SMS with ASP.NET MVC Application:
I have already defined TheTexting API and its key parameters in the previous section. In addition to that, I have also attached a demo for a web application. Keep in mind that before you can use their gateway to send SMS, you need to sign up for an account with thetexting.com to get your API key and Secret values.
http://www.thetexting.com
Software Requirements to run the demo for MVC:
? Visual studio 2015 must install on your machine.
? You must have MVC 5 installed with Visual studio
? Newtonsoft.Json.dll reference need to be added
How to open the attached project in your Visual studio below are the steps:
? Download the project and extract it to your desired folder.
? Open up visual studio with administrator rights.
? Click on File Menu then select an open project from the menu a window will pop up find the location where you unzipped the project and select TheTextingDemo.sln and click open.
? This is a pre-templated MVC project I just have added a simple class in the model's folder with name ConfigurationSettings.cs to set the configuration like API KYE, SECRET, TO, MESSAGE etc.
? I have just modified the index.cshtml view and home Controller.
Our class definition is like below
usingSystem.Linq;
usingSystem.Web;
namespaceTheTextingDemo.Models
{
publicclassConfigurationSettings
{
[Key]
[ScaffoldColumn(false)]
publicintRecordID { get; set; }
[Required(ErrorMessage = "API_KEY is required")]
[Display(Name = "Enter API_KEY Name")]
publicstring API_KEY { get; set; }
[Required(ErrorMessage = "API_SECRET is required")]
publicstring API_SECRET { get; set; }
[Required(ErrorMessage = "To_Number is required")]
[Display(Name = "To_Number")]
publicdoubleTo_Number { get; set; }
//[DataType(DataType.PhoneNumber)]
[Required(ErrorMessage = "Message is required")]
publicstringTextMessage { get; set; }
}
}
For the sake of simplicity, I will not drag the article out by defining each and everything related to MVC. If you want to learn MVC first than follow the link below
https://www.asp.net/mvc/overview HYPERLINK "https://www.asp.net/mvc/overview".
Index.cshtml:
@model TheTextingDemo.Models.ConfigurationSettings
<h2>TheTexting SMS GateWay Demo</h2>
<style type="text/css">
.required:after {
padding-left: 10px;
content: "*";
font-weight: bold;
color: red;
}
</style>
<script src="@Url.Content("~/Scripts/jquery.validate.min.js")" type="text/javascript"></script>
<script src="@Url.Content("~/Scripts/jquery.validate.unobtrusive.min.js")" type="text/javascript"></script>
@Html.ValidationSummary(true, "Correct The Errors first.")
@using (Html.BeginForm("SendSMS", "Home", FormMethod.Post))
{
<div>
<fieldset>
<legend>Account Information</legend>
<div>
<h3>Note: all the fields with (*) are required fields.</h3>
</div>
<div>
@Html.LabelFor(model => model.API_KEY, new { @class = "required" })
</div>
<div>
@Html.TextBoxFor(model => model.API_KEY)
@Html.ValidationMessageFor(model => model.API_KEY)
</div>
<div>
@Html.LabelFor(model => model.API_SECRET, new { @class = "required" })
</div>
<div>
@Html.TextBoxFor(model => model.API_SECRET)
@Html.ValidationMessageFor(model => model.API_SECRET)
</div>
<div>
@Html.LabelFor(a => a.To_Number, new { @class = "required" })
</div>
<div>
@Html.TextBoxFor(a => a.To_Number)
@Html.ValidationMessageFor(a => a.To_Number)
</div>
<div>
@Html.LabelFor(a => a.TextMessage, new { @class = "required" })
</div>
<div>
@Html.TextAreaFor(a => a.TextMessage, new { @Value = "test" })
@Html.ValidationMessageFor(a => a.TextMessage)
</div>
<br />
<input id="Submit" type="submit" value="SendSMS" />
<br /><br />
<h4 style="display:inline; color:red;">
Note:
<p style="display:inline;">Number must contain the country code like for us we have 1-3306807200</p>
</h4>
</fieldset>
</div>
}
I made my view as strongly typed view as @model TheTextingDemo.Models.ConfigurationSettings
because I want to get access the values of the textboxes placed in the view into my controller.Next I create a method in the home controller with [httppost] attribute because I want to post these values on button click event , our controller code look like this as shown below.
home Controller:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using System.IO;
using System.Net;
using System.Web.UI.WebControls;
using TheTextingDemo.Models;
namespace TheTextingDemo.Controllers
{
public class HomeController: Controller
{
public class Response
{
public string message_id { get; set; }
public int message_count { get; set; }
public double price { get; set; }
}
public class RootObject
{
public Response Response { get; set; }
public string ErrorMessage { get; set; }
public int Status { get; set; }
}
public ActionResult Index()
{
return View();
}
[HttpPost]
public ActionResult SendSMS(ConfigurationSettings sm)
{
string API_KEY = sm.API_KEY;
string API_SECRET = sm.API_SECRET;
double TO = Convert.ToDouble(sm.To_Number);
string Message = sm.TextMessage;
string sURL;
sURL = "https://www.thetexting.com/rest/sms/json/message/send?api_key=" + API_KEY + "&api_secret=" + API_SECRET + "&to=" + TO + "&text=" + Message;
if (ModelState.IsValid)
{
try
{
using (WebClient client = new WebClient())
{
string s = client.DownloadString(sURL);
var responseObject = Newtonsoft.Json.JsonConvert.DeserializeObject<RootObject>(s);
int n = responseObject.Status;
if (n == 3)
{
return Content("<script>alert('Message does not Send Successfully due to invalid credentials !');location.href='/';</script>");
}
else
{
return Content("<script>alert('Message Send Successfully !');location.href='/';</script>");
}
}
}
catch (Exception ex)
{
ModelState.AddModelError("", "Error in sending Message");
ex.ToString();
}
return View("Index");
}
else
{
ModelState.AddModelError("", "Error in sending Message");
return View("Index");
}
}
}
}
There is no difference in the C# code just the structure of windows application vs web applications differs when you run the project the following screen will appears.
http://imgur.com/a/mlAkD
Replace the API_KEY and API_SECRET Values with the ones you have given after creation of your account with theTexting.com and hit sendSMS button.
Source Code Files
I have attached source code files for both windows and the web:
? Windows example- TheTextingDemoAppWindows.zip
? Web MVC example- TheTextingDemo MVC.zip
you can download the demo from dropbox link below due to the size of the files i am unable to push them here.
Conclusion
Presently you can respite and slow down. I trust you didn't lose all sense of direction in my clarifications. In the event that you have taken after the means nearly, you ought to have the capacity to send SMS utilizing C# application with thetexting.com without much trouble. I did my absolute best to make it basic and to walk you through every one of the points of interest as easily as could be expected under the circumstances. I trust this article will be useful to everyone from beginners to advance level.
References
The texting
Drop Box Link:
https://www.dropbox.com/sh/ezlxh722srf8zgl/AAA-sB4nBb29i_x9ZexkE8FGa?dl=0
By Ahmed
Introduction:
In this article, I will walk you through a fast and intriguing approach to send SMS utilizing straightforward C #applications. I make it as simple as feasible for you so I have made both the windows and web demo applications so as to show the guidelines that I will clarify in this article. So simply after taking these simple strides as I walk you through the entire procedure and in the blink of an eye sending SMS will be easy for you.
As cell phones have moved toward becoming a vital part of our regular daily existences and none of us can manage without them anymore, it is not a big surprise that measurements have demonstrated that SMS is the best approach to impact, particularly with regards to a business correspondence. Considering this I set out to demonstrate to you a simple approach to sending SMS using C#.
I will utilize TheTexting SMS API to send SMS, since there are numerous different SMS entryways, you can experiment with any of them by utilizing their free trial account. I could not find any article showing sending SMS using TheTexting gateway, that force me to write an article on sending SMS via TheTexting SMS gateway.
Different ways of sending text messages?
There are different ways of sending text messages like below?
1-Using GSM Modem:
This would be the best decision in the event that you need to send SMS from disconnected applications and in the event that you need to send few SMS for consistently then utilizing GSM modem is the best decision.
2-Using endpoints:
It is the best decision when the quantity of SMS should have been sent surpasses a hundred every moment.
3-Using web service:
This would be the best decision for an online application and you want to send very few messages every minute.
Sending SMS via web service:
We should begin without delving further into the superfluous insights about different SMS API providers in the market. Going into profundities at this stage will just make it additionally befuddling and it is not my expectation to give you a migraine. I will explain the procedure well-ordered with the goal that you can digest easily.
Part one:
Get Started:
The first thing you need to do is to create an account on http://www.thetexting.com so what you need to do is to click on this link while holding the CTRL key of your keyboard. You can also copy and paste the link into your browser window and hit enter key of your keyboard. You will see the below screen.
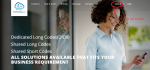
By clicking on the sign up link you will be redirected to the following page as show in below figure
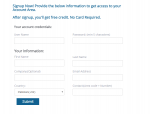
You have to fill out this form with the required information and submit it, after submission of the form you will received SMS as well as email from TheTexting,com and you have to verify both of them before using TheTexting SMS gateway. You can see in the figure bellow how it looks like.
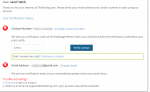
After verification of your phone number and email you would be redirected to the following page as shown below.
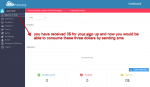
This Dashboard gives you the overall details like your balance, API Settings, profile, and developer references, the one which is of our interest is API Settings, we can generate new API key as well by clicking the Generate API Key button. Just click on API setting and it will show your key and secret values as shown below in figure.
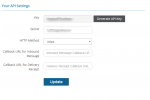
Service URL:
https://www.thetexting.com/rest/sms/json/message/send?api_key=wwpiub1oipk49a1&api_secret=j5hsuac8877mdzdd&to=923218829981&text=Hello"
This is the API service URL which our Web client will be consuming. We just need to change the values of API KEY , SECRET and TO values and hit enter the SMS will be sent to the number which you specified in the To parameter. Is not that a magic without creating any application just with a URL you are able to send SMS? Wow
Now, let?s start with the demo application I am creating WPF application for demonstration for windows platform , if you are unfamiliar with WPF then go to the below link to learn WPF I do not want to spin my article out to teach you WPF first.
https://msdn.microsoft.com/en-us/library/aa970268(v=vs.100).aspx
Here we have four textboxes for four input parameters like API key, secret, To and Message, I have already demonstrated these four parameters above. To get your API key and secret values just login to http://www.thetexting.com and then click on API settings here you will find your API key and Secret values, To is the number to which you want to send SMS and do not forget to include country Code like I did for United sates it is 1. Message is the message which you want to send, due to trial account you cannot provide a custom message TheTexting will send the default message same as you cannot provide the from parameter input value as it also would be by default thetexting itself.
C # code for sending SMS:
public class Response
{
public string message_id { get; set; }
public int message_count { get; set; }
public double price { get; set; }
}
public class RootObject
{
public Response Response { get; set; }
public string ErrorMessage { get; set; }
public int Status { get; set; }
}
private void button1_Click(object sender, RoutedEventArgs e)
{
string key = txtKey.Text;
string secret = txtSecret.Text;
string to = txtTo.Text;
string messages = txtMessage.Text;
string sURL;
sURL = "https://www.thetexting.com/rest/sms/json/message/send?api_key=" + key + "&api_secret=" + secret + "&to=" + to + "&text=" + messages;
try
{
using (WebClient client = new WebClient())
{
string s = client.DownloadString(sURL);
var responseObject = Newtonsoft.Json.JsonConvert.DeserializeObject<RootObject>(s);
int n=responseObject.Status;
if (n == 3)
{
MessageBox.Show("Something went wrong with your credentials", "My Demo App");
}
else
{
MessageBox.Show("Message Send Successfully", "My Demo App");
}
}
}
catch (Exception ex)
{
MessageBox.Show("Something went wrong with your information", "My Demo App");
ex.ToString();
}
}
}
Here we have a very simple code. We have created four variables and we are assigning the four text boxes values to these four variables. Next we are creating a string variable of URL and giving it our service URL and including our parameters values with it i.e. SECRET,API KEY , TO and Message. With this URL{this URL is basically the address of our service}, then we are using web client to download the data, our service will return data in JSON format so now we want to read this JSON result using our c# code,for that we again have two options to do that, one method is to read data from datatable by first pulling the data into the data table and then reading from it, but the best approach is to deserialize JSON data, so we have created two class Response and RootObject and they have their own properties like message id,price,count,status, then we are using Newtonsoft.Json.JsonConvert.DeserializeObject<RootObject>(s) to convert the JSON data to C# and reading the status value to show the specified message to the user i.e. if the status code is 3 then it means that authentication failed and based on these codes we are showing specific messages to the user on screen as message pop-up.
If you need details about how to Deserialize JSON Data then visit below link.
http://stackoverflow.com/questions/7895105/deserialize-json-with-c-sharp
Namespaces Required:
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using System.IO;
using System.Net;
using System.Data;
using System.Xml;
using Newtonsoft.Json;
Note: you must include Newtonsoft.Json.dll into your references, the demo application having already included Newtonsoft.Json.dll but for double check, I have also placed it in zip folder along with the attachments.
Part Two:
How to send SMS with ASP.NET MVC Application:
I have already defined TheTexting API and its key parameters in the previous section. In addition to that, I have also attached a demo for a web application. Keep in mind that before you can use their gateway to send SMS, you need to sign up for an account with thetexting.com to get your API key and Secret values.
http://www.thetexting.com
Software Requirements to run the demo for MVC:
? Visual studio 2015 must install on your machine.
? You must have MVC 5 installed with Visual studio
? Newtonsoft.Json.dll reference need to be added
How to open the attached project in your Visual studio below are the steps:
? Download the project and extract it to your desired folder.
? Open up visual studio with administrator rights.
? Click on File Menu then select an open project from the menu a window will pop up find the location where you unzipped the project and select TheTextingDemo.sln and click open.
? This is a pre-templated MVC project I just have added a simple class in the model's folder with name ConfigurationSettings.cs to set the configuration like API KYE, SECRET, TO, MESSAGE etc.
? I have just modified the index.cshtml view and home Controller.
Our class definition is like below
usingSystem.Linq;
usingSystem.Web;
namespaceTheTextingDemo.Models
{
publicclassConfigurationSettings
{
[Key]
[ScaffoldColumn(false)]
publicintRecordID { get; set; }
[Required(ErrorMessage = "API_KEY is required")]
[Display(Name = "Enter API_KEY Name")]
publicstring API_KEY { get; set; }
[Required(ErrorMessage = "API_SECRET is required")]
publicstring API_SECRET { get; set; }
[Required(ErrorMessage = "To_Number is required")]
[Display(Name = "To_Number")]
publicdoubleTo_Number { get; set; }
//[DataType(DataType.PhoneNumber)]
[Required(ErrorMessage = "Message is required")]
publicstringTextMessage { get; set; }
}
}
For the sake of simplicity, I will not drag the article out by defining each and everything related to MVC. If you want to learn MVC first than follow the link below
https://www.asp.net/mvc/overview HYPERLINK "https://www.asp.net/mvc/overview".
Index.cshtml:
@model TheTextingDemo.Models.ConfigurationSettings
<h2>TheTexting SMS GateWay Demo</h2>
<style type="text/css">
.required:after {
padding-left: 10px;
content: "*";
font-weight: bold;
color: red;
}
</style>
<script src="@Url.Content("~/Scripts/jquery.validate.min.js")" type="text/javascript"></script>
<script src="@Url.Content("~/Scripts/jquery.validate.unobtrusive.min.js")" type="text/javascript"></script>
@Html.ValidationSummary(true, "Correct The Errors first.")
@using (Html.BeginForm("SendSMS", "Home", FormMethod.Post))
{
<div>
<fieldset>
<legend>Account Information</legend>
<div>
<h3>Note: all the fields with (*) are required fields.</h3>
</div>
<div>
@Html.LabelFor(model => model.API_KEY, new { @class = "required" })
</div>
<div>
@Html.TextBoxFor(model => model.API_KEY)
@Html.ValidationMessageFor(model => model.API_KEY)
</div>
<div>
@Html.LabelFor(model => model.API_SECRET, new { @class = "required" })
</div>
<div>
@Html.TextBoxFor(model => model.API_SECRET)
@Html.ValidationMessageFor(model => model.API_SECRET)
</div>
<div>
@Html.LabelFor(a => a.To_Number, new { @class = "required" })
</div>
<div>
@Html.TextBoxFor(a => a.To_Number)
@Html.ValidationMessageFor(a => a.To_Number)
</div>
<div>
@Html.LabelFor(a => a.TextMessage, new { @class = "required" })
</div>
<div>
@Html.TextAreaFor(a => a.TextMessage, new { @Value = "test" })
@Html.ValidationMessageFor(a => a.TextMessage)
</div>
<br />
<input id="Submit" type="submit" value="SendSMS" />
<br /><br />
<h4 style="display:inline; color:red;">
Note:
<p style="display:inline;">Number must contain the country code like for us we have 1-3306807200</p>
</h4>
</fieldset>
</div>
}
I made my view as strongly typed view as @model TheTextingDemo.Models.ConfigurationSettings
because I want to get access the values of the textboxes placed in the view into my controller.Next I create a method in the home controller with [httppost] attribute because I want to post these values on button click event , our controller code look like this as shown below.
home Controller:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using System.IO;
using System.Net;
using System.Web.UI.WebControls;
using TheTextingDemo.Models;
namespace TheTextingDemo.Controllers
{
public class HomeController: Controller
{
public class Response
{
public string message_id { get; set; }
public int message_count { get; set; }
public double price { get; set; }
}
public class RootObject
{
public Response Response { get; set; }
public string ErrorMessage { get; set; }
public int Status { get; set; }
}
public ActionResult Index()
{
return View();
}
[HttpPost]
public ActionResult SendSMS(ConfigurationSettings sm)
{
string API_KEY = sm.API_KEY;
string API_SECRET = sm.API_SECRET;
double TO = Convert.ToDouble(sm.To_Number);
string Message = sm.TextMessage;
string sURL;
sURL = "https://www.thetexting.com/rest/sms/json/message/send?api_key=" + API_KEY + "&api_secret=" + API_SECRET + "&to=" + TO + "&text=" + Message;
if (ModelState.IsValid)
{
try
{
using (WebClient client = new WebClient())
{
string s = client.DownloadString(sURL);
var responseObject = Newtonsoft.Json.JsonConvert.DeserializeObject<RootObject>(s);
int n = responseObject.Status;
if (n == 3)
{
return Content("<script>alert('Message does not Send Successfully due to invalid credentials !');location.href='/';</script>");
}
else
{
return Content("<script>alert('Message Send Successfully !');location.href='/';</script>");
}
}
}
catch (Exception ex)
{
ModelState.AddModelError("", "Error in sending Message");
ex.ToString();
}
return View("Index");
}
else
{
ModelState.AddModelError("", "Error in sending Message");
return View("Index");
}
}
}
}
There is no difference in the C# code just the structure of windows application vs web applications differs when you run the project the following screen will appears.
http://imgur.com/a/mlAkD
Replace the API_KEY and API_SECRET Values with the ones you have given after creation of your account with theTexting.com and hit sendSMS button.
Source Code Files
I have attached source code files for both windows and the web:
? Windows example- TheTextingDemoAppWindows.zip
? Web MVC example- TheTextingDemo MVC.zip
you can download the demo from dropbox link below due to the size of the files i am unable to push them here.
Conclusion
Presently you can respite and slow down. I trust you didn't lose all sense of direction in my clarifications. In the event that you have taken after the means nearly, you ought to have the capacity to send SMS utilizing C# application with thetexting.com without much trouble. I did my absolute best to make it basic and to walk you through every one of the points of interest as easily as could be expected under the circumstances. I trust this article will be useful to everyone from beginners to advance level.
References
The texting
Drop Box Link:
https://www.dropbox.com/sh/ezlxh722srf8zgl/AAA-sB4nBb29i_x9ZexkE8FGa?dl=0