NiceGirl13
New member
- Joined
- Nov 19, 2015
- Messages
- 4
- Programming Experience
- Beginner
I have created a WPF project, where a user can insert the Servername in a textbox, and the connection should be saved in the app.config (in Runtime), ones that is done the data from a DB table should be loaded in the datagrid. When i press the connect button (after inserted the servername in the textbox) i get this message:
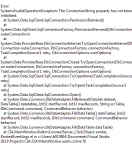
The code is as followed:
App.config
MainWindow.xaml.cs
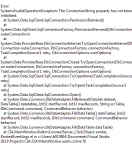
The code is as followed:
App.config
C#:
<configuration>
<startup>
<supportedRuntime version="v4.0" sku=".NETFramework,Version=v4.5" />
</startup>
<connectionStrings>
<add name="SQLconnectionString"
connectionString=""
providerName="System.Data.sqlclient"/>
</connectionStrings>
</configuration>
MainWindow.xaml.cs
C#:
namespace CIA
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void ButtonConnectServer_Click(object sender, RoutedEventArgs e)
{
try
{
StringBuilder Con = new StringBuilder("Server=");
Con.Append(TextBoxServerName.Text);
Con.Append("; Database=SIT-DVH; Trusted_Connection=True;");
string strCon = Con.ToString();
updateConfigFile(strCon);
SqlConnection db = new SqlConnection();
ConfigurationManager.RefreshSection("connectionStrings");
db.ConnectionString = ConfigurationManager.ConnectionStrings["SQLconnectionString"].ToString();
// if connect to the server and database is there, load values into the datagrid
SqlDataAdapter sda = new SqlDataAdapter("SELECT * FROM Customer_Information",db);
DataTable data = new DataTable();
sda.Fill(data);
DataGridView_Customer_Information.DataContext = data;
//or change to this:
//DataGridView_Customer_Information.ItemsSource = data.DefaultView;
}
catch (Exception ex)
{
MessageBox.Show("Error: \r\n" + ex);
}
}
public void updateConfigFile(string con)
{
//Updating config file
XmlDocument XmlDoc = new XmlDocument();
//Loading the config file
XmlDoc.Load(AppDomain.CurrentDomain.SetupInformation.ConfigurationFile);
foreach (XmlElement xElement in XmlDoc.DocumentElement)
{
if (xElement.Name == "connectionStrings")
{
//setting the connectionStrings
xElement.FirstChild.Attributes[2].Value = SQLconnectionString;
}
}
//writing the connection string into the config file
XmlDoc.Save(AppDomain.CurrentDomain.SetupInformation.ConfigurationFile);
}
}
}
Last edited: